Reading from a file in Node.js
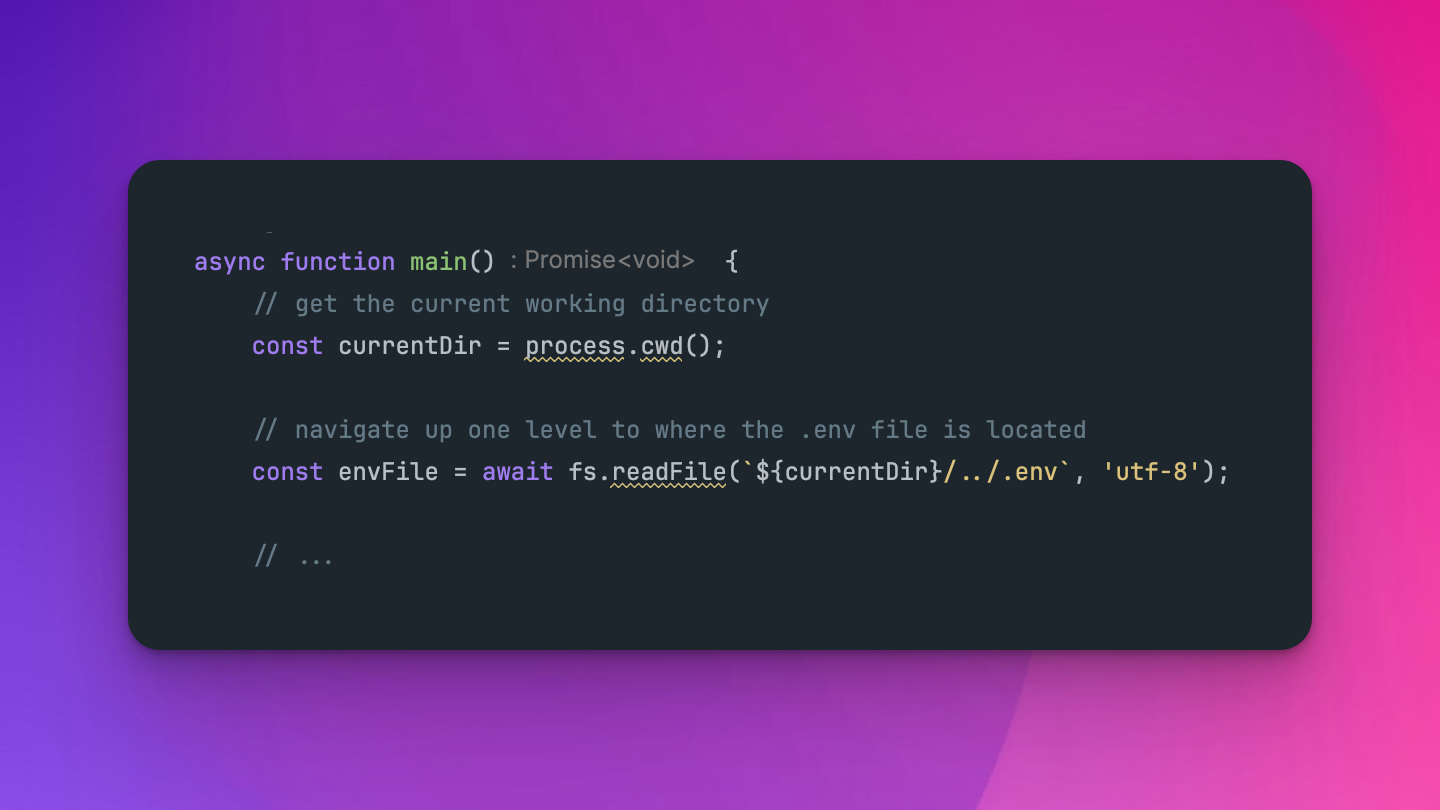
In this lightning tutorial, we'll walk through what it takes to read a file using Node.js, using a practical, real-world example where our basic app will read from a configuration file (a .env
file in this case).
0. Install Node.js
There are many different ways to install Node.js on your machine. I personally use NVM to manage multiple node versions on my machine with ease, but it's really up to you. Some of your options include:
- Direct download from the official Node.js site: https://nodejs.org/en/download
- Configure Node Version Manager (NVM) and install: https://github.com/nvm-sh/nvm
- Install using Homebrew (MacOS) by running:
brew install node
- Install from source code
- ...and more!
To ensure you have installed Node.js on your machine successfully, from your command line, run: node -v
which will output the current verions of Node.js installed on your machine.
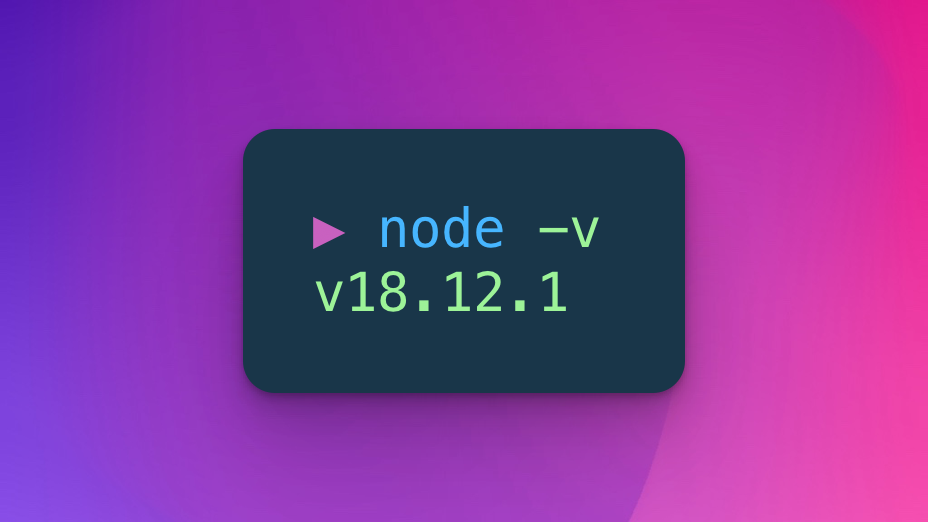
1. Setup the project structure
Once you have installed Node.js, let's configure the basic project structure for our tutorial. We'll use a more conventional project structure where the app code lives in a src/
directory, and other artifacts and config files such as the .env
file live outside of the src/
directory.
# create a directory
mkdir node-read-file
# change into the new directory
cd node-read-file
# create src dir
mkdir src
# create .env file
touch .env
# change into src dir
cd src
# create main app file
touch app.js
2. Add environment variables to .env
file
In today's day and age, a lot of apps – either knowingly or unknowingly – adhere to the principles outlined in "The Twelve-Factor App".
The 3rd factor of a Twelve-Factor App is "Config", which is essentially anything that will vary between deploys. For example, the database your application connects to during local development would (and should!) likely be different than the database your app uses in production. Database credentials are almost always a good candidate to store in configuration, such a .env
file.
Add these environment variables to your .env
:
DATABASE_HOST=localhost
DATABASE_PORT=5432
DATABASE_NAME=codesnippet
DATABASE_USER=codesnippet_user
DATABASE_PASSWORD=passw0rd1234!
3. Read the .env
file in src/app.js
Now that we have setup our .env
file with some environment variables, we can now read the .env
in our application, and use the environment variables as needed.
In the src/app.js
file, let's add some code:
const fs = require('node:fs/promises');
async function main() {
// get the current working directory
const currentDir = process.cwd();
// navigate up one level to where the .env file is located
const envFile = await fs.readFile(`${currentDir}/../.env`, 'utf-8');
// parse the .env file into a javascript object
const env = convertEnvFileToObject(envFile);
// connect to our dummy database
// passing in the environment variables as parameters
connectToDatabase({
host: env.DB_HOST,
port: env.DB_PORT,
name: env.DB_NAME,
user: env.DB_USER,
password: env.DB_PASSWORD,
});
}
function connectToDatabase({ host, port, name, user, password }) {
// check that all the required parameters are present
if (!host || !port || !name || !user || !password) {
throw new Error('Invalid database configuration');
}
console.log(`Successfully connected to database: ${user}:${password}@${host}:${port}/${name}`);
}
function convertEnvFileToObject(file) {
// split the file into lines
const lines = file.split('\n');
// create an empty object to store the parsed values
const parsed = {};
// iterate over each line of the file
for (const line of lines) {
// skip comments
if (line.startsWith('#')) {
continue;
}
// split the line into key and value
const [key, value] = line.split('=');
// store the key and value in the object
parsed[key] = value;
}
// return the parsed object
return parsed;
}
main().catch((err) => console.error(err));
It's worth noting that the reading of the file itself occurs in essentially one line of code, where we call fs.readFile()
which is an asynchronous operation that returns the contents of the specified file.
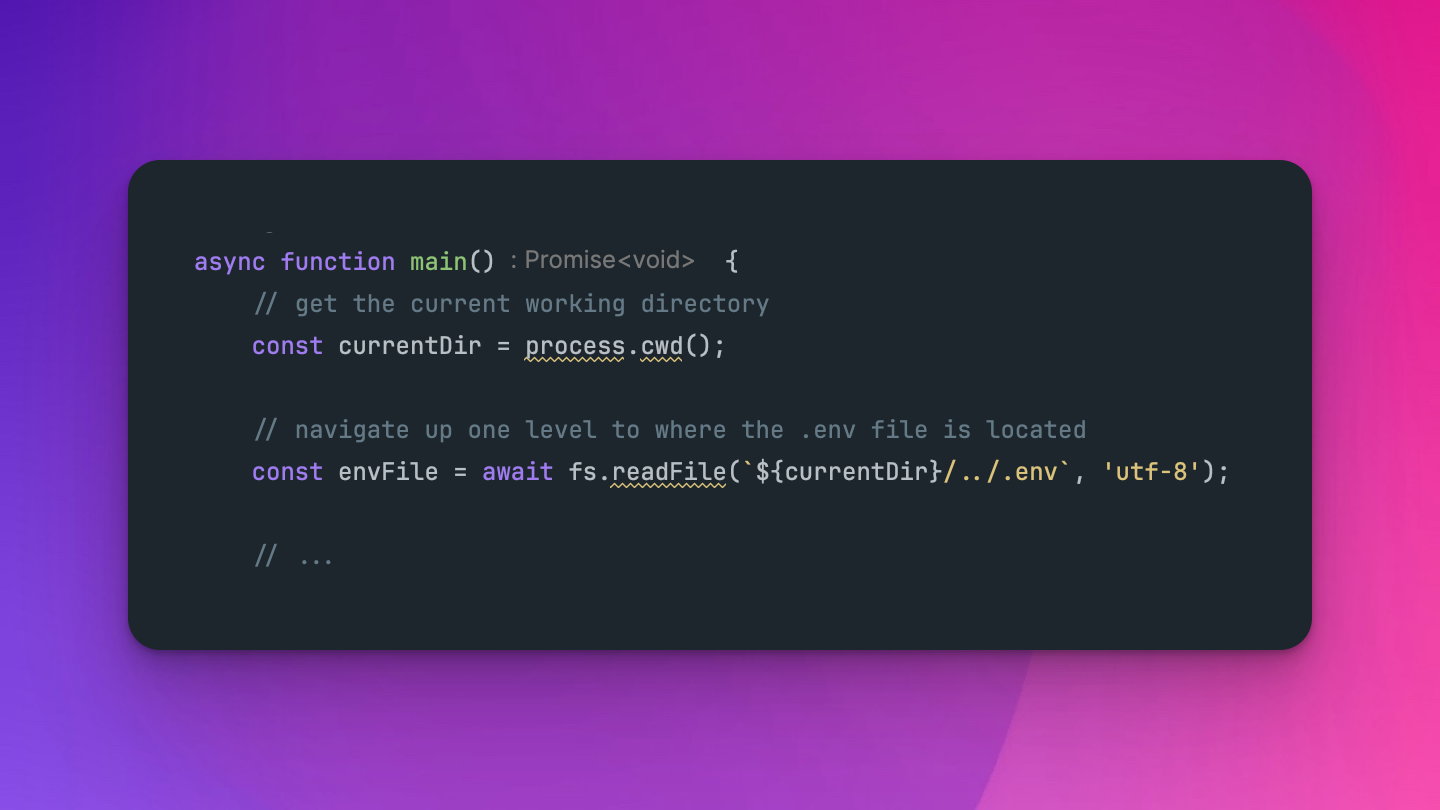
fs.readFile()
API.4. Running the app
The last thing left for us to do is to run our application, we can do this from the command line. Make sure you're in the root directory of you project, and the run node src/app.js
. You should then see log output indicating that our application successfully read from the .env
file using the fs.readFile()
API, and connected to our dummy "database"!
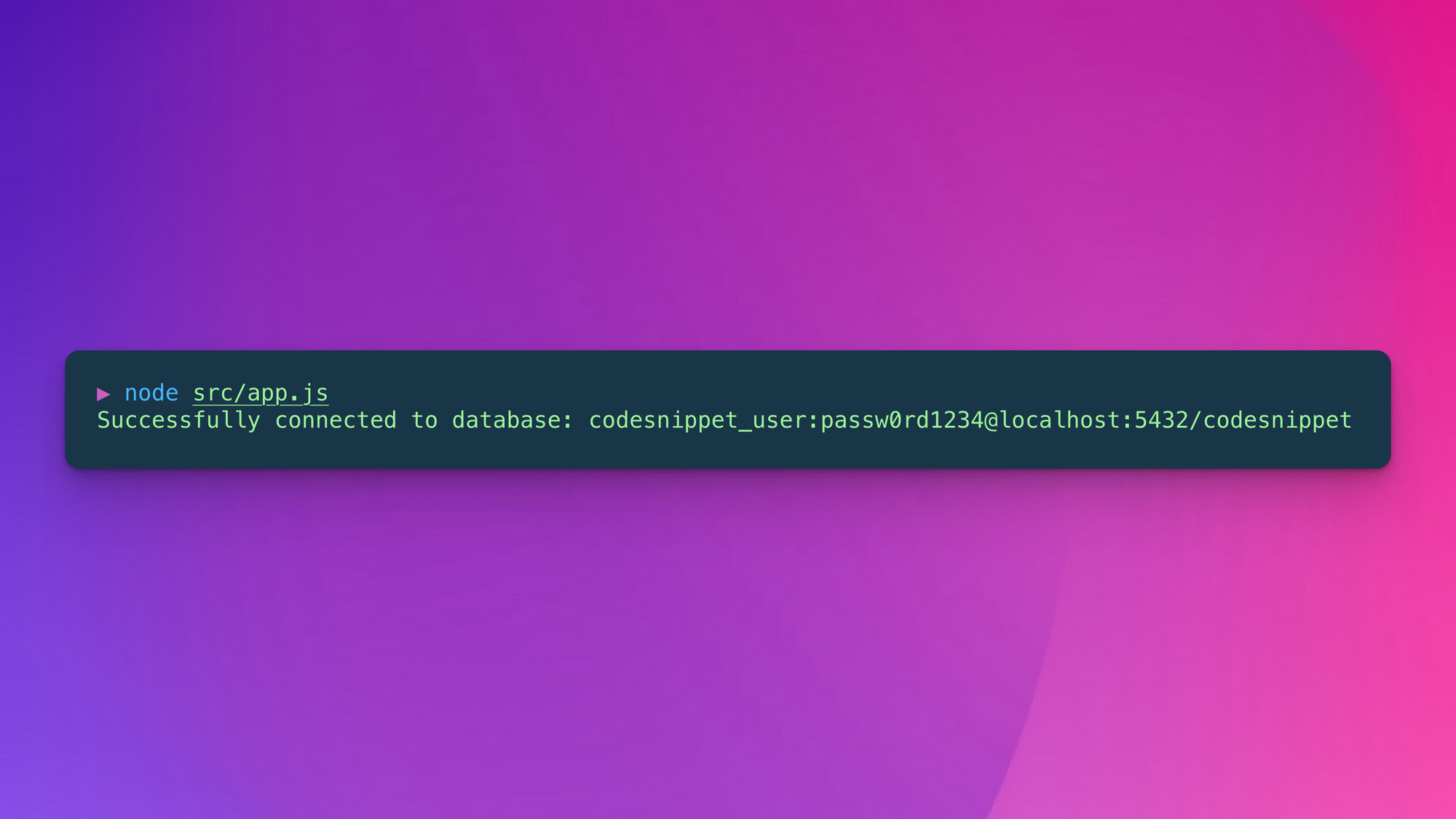
Help us improve our content